Documentation
Resolving jQWidgets Script Dependencies with RequireJS

The scripts in a jQWidgets project have dependencies to jQuery, jqxcore.js
and often other jQWidgets scripts. In this tutorial you will learn how to resolve
jQWidgets script dependencies with RequireJS. Before you proceed further, make sure
you are familiar with the basic jQWidgets
Integration with RequireJS tutorial. The example that will be showcased
here is based on the jqxGrid demo Spreadsheet.
1. Create a Project
As with the basic tutorial,
we will create a "project", consisting of an .html
page (index.html
)
and required CSS and JavaScript files (require.js
, jquery.js
,
the necessary jQWidgets scripts, main.js
and app.js
).
Here is the folder structure of our project:
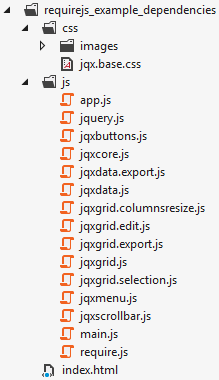
2. index.html
Here is the code of the file index.html
:
<!DOCTYPE html><html lang="en"><head><title>Example of Resolving jQWidgets Script Dependencies with RequireJS</title><link rel="Stylesheet" href="css/jqx.base.css" type="text/css" /><script type="text/javascript" data-main="js/main" src="js/require.js"></script></head><body class='default'><div id='jqxWidget'><div id="jqxgrid"></div><div style='margin-top: 20px;'><div style='float: left;'><input type="button" value="Export to Excel" id='excelExport' /></div></div></div></body></html>
The code includes a reference to the file require.js
, whose data-main
attribute points to main.js
and the required data-bound HTML structure.
More on main.js
in Step 3.
3. main.js
and app.js
Here is the code of the script main.js
:
require.config({paths: {"jquery": "jquery","jqxcore": "jqxcore","jqxdata": "jqxdata","jqxdata.export": "jqxdata.export","jqxgrid": "jqxgrid","jqxgrid.columnsresize": "jqxgrid.columnsresize","jqxgrid.edit": "jqxgrid.edit","jqxgrid.export": "jqxgrid.export","jqxgrid.selection": "jqxgrid.selection","jqxbuttons": "jqxbuttons","jqxscrollbar": "jqxscrollbar","jqxmenu": "jqxmenu"},shim: {"jqxcore": {export: "$",deps: ["jquery"]},"jqxdata": {export: "$",deps: ["jquery", "jqxcore"]},"jqxdata.export": {export: "$",deps: ["jquery", "jqxcore", "jqxdata"]},"jqxgrid": {export: "$",deps: ["jquery", "jqxcore", "jqxdata"]},"jqxgrid.columnsresize": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]},"jqxgrid.edit": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]},"jqxgrid.export": {export: "$",deps: ["jquery", "jqxcore", "jqxdata.export", "jqxgrid"]},"jqxgrid.selection": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]},"jqxbuttons": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]},"jqxscrollbar": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]},"jqxmenu": {export: "$",deps: ["jquery", "jqxcore", "jqxgrid"]}}});require(["app"], function (App) {App.initialize();});
The shim
configuration object is essential for the correct loading
order of the scripts (modules). Each script in shim
has the the scripts
it depends on defined in the deps
array. The script main.js
also loads the application module (the web page functionality, located in app.js
):
And here is the code of app.js
:
define(["jquery", "jqxcore", "jqxdata", "jqxdata.export", "jqxgrid", "jqxgrid.columnsresize", "jqxgrid.edit", "jqxgrid.export", "jqxgrid.selection", "jqxbuttons", "jqxscrollbar", "jqxmenu"], function () {var initialize = function () {$(document).ready(function () {// renderer for grid cells.var numberrenderer = function (row, column, value) {return '<div style="text-align: center; margin-top: 5px;">' + (1 + value) + '</div>';}// create Grid datafields and columns arrays.var datafields = [];var columns = [];for (var i = 0; i < 26; i++) {var text = String.fromCharCode(65 + i);if (i == 0) {var cssclass = 'jqx-widget-header';columns[columns.length] = { pinned: true, exportable: false, text: "", columntype: 'number', cellclassname: cssclass, cellsrenderer: numberrenderer };}datafields[datafields.length] = { name: text };columns[columns.length] = { text: text, datafield: text, width: 60, align: 'center' };}var source ={unboundmode: true,totalrecords: 100,datafields: datafields,updaterow: function (rowid, rowdata) {// synchronize with the server - send update command}};var dataAdapter = new $.jqx.dataAdapter(source);// initialize jqxGrid$("#jqxgrid").jqxGrid({width: 670,source: dataAdapter,editable: true,columnsresize: true,selectionmode: 'multiplecellsadvanced',columns: columns});$("#excelExport").jqxButton();$("#excelExport").click(function () {$("#jqxgrid").jqxGrid('exportdata', 'xls', 'jqxGrid', false);});});};return {initialize: initialize};});
All jQWidgets scripts and jQuery should be included in an array, which is passed
as the first argument of the RequireJS define
function. The second
argument is a callback function. Its code is based on the aforementioned demo Spreadsheet.
4. Download the Example or Try It Out
You can download the complete example from here or check it online from here: Example of Resolving jQWidgets Script Dependencies with RequireJS.