Angular UI Components
Introduction
Build modern Web Apps with jQWidgets and Angular. jQWidgets Angular UI components will help you to build perfect looking web applications. Use the most advanced UI framework for Angular and save your time for the business logic. Choose from more than 60 UI components for Angular.
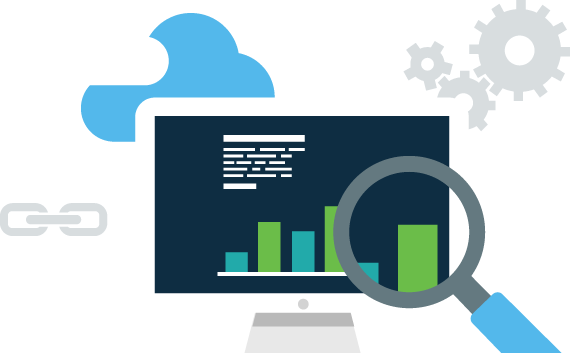

Highlights
Angular is a development platform for building mobile and desktop web applications. It provides a way to build apps for any deployment target by reusing existing code. Using HTML as the template language, Angular offers developers the possiblity to create their own components. We created multiple components by using Angular, Typescript and the jQWidgets framework. The Typescript files for all Angular UI components are located in the jqwidgets-ts folder of the download package.

Getting Started
Getting Started documentation about using our components is available on: jQWidgets Components for Angular
Browse our demos to see what you can do and how to use our product.Quick Start
The goal in this guide is to build and run a simple Angular application in TypeScript, using the Angular CLI and jQWidgets.
Step 1. Set up the Development Environment
Install Node.js and npm if they are not already on your machine.
Verify that you are running at least Node.js version 8.x or greater and npm version 5.x or greater by running node -v and npm -v in a terminal/console window.
Then install the Angular CLI globally.
npm install -g @angular/cli
Step 2. Create a new project
Open a terminal window.
Generate a new project and default app by running the following command:
ng new my-app
The Angular CLI installs the necessary npm packages, creates the project files, and populates the project with a simple default app. This can take some time.
Step 3: Serve the application
Go to the project directory.
cd my-app
Install the jqwidgets-ng dependency
npm install jqwidgets-ng
Open the angular.json file and inside the styles property add this line:
"node_modules/jqwidgets-ng/jqwidgets/styles/jqx.base.css"
Launch the server.
ng serve --open
The ng serve command launches the server, watches your files, and rebuilds the app as you make changes to those files.
Using the --open (or just -o) option will automatically open your browser on http://localhost:4200/.
Your app greets you with a message.
Step 4: Edit your first Angular component
The CLI created the first Angular component for you. This is the root component and it is named app-root. You can find it in ./src/app/app.component.ts. We will use jqxBarGaugeComponent so we will add a property named values
.
Open the component file and replace the code with the code below.
src/app/app.component.ts
@Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { values: number[] = [102, 115, 130, 137]; }
src/app/app.component.html
Open src/app/app.component.html and give the component the following html
<jqxBarGauge [width]="600" [height]="600" [max]="150" [colorScheme]="'scheme02'" [values]="values"></jqxBarGauge>
src/app/app.module.ts
Open src/app/app.module.ts and import jqxBarGaugeComponent
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { jqxBarGaugeModule } from 'jqwidgets-ng/jqxbargauge'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, jqxBarGaugeModule ], bootstrap: [ AppComponent ] }) export class AppModule {}
Result is displayed on: localhost:4200

Project File Overview
File | Purpose |
---|---|
|
Defines the |
|
Defines |
|
A folder where you can put images and anything else to be copied wholesale when you build your application. |
|
This folder contains one file for each of your destination environments, each exporting simple configuration variables to use in your application. The files are replaced on-the-fly when you build your app. You might use a different API endpoint for development than you do for production or maybe different analytics tokens. You might even use some mock services. Either way, the CLI has you covered. |
|
A configuration file to share target browsers between different front-end tools. |
|
Every site wants to look good on the bookmark bar. Get started with your very own Angular icon. |
|
The main HTML page that is served when someone visits your site.
Most of the time you'll never need to edit it.
The CLI automatically adds all |
|
Unit test configuration for the Karma test runner,
used when running |
|
The main entry point for your app.
Compiles the application with the JIT compiler
and bootstraps the application's root module ( |
|
Different browsers have different levels of support of the web standards.
Polyfills help normalize those differences.
You should be pretty safe with |
|
Your global styles go here. Most of the time you'll want to have local styles in your components for easier maintenance, but styles that affect all of your app need to be in a central place. |
|
This is the main entry point for your unit tests. It has some custom configuration that might be unfamiliar, but it's not something you'll need to edit. |
|
TypeScript compiler configuration for the Angular app ( |
|
Additional Linting configuration for TSLint together with
Codelyzer, used when running |
|
Inside |
|
|
|
Simple configuration for your editor to make sure everyone that uses your project
has the same basic configuration.
Most editors support an |
|
Git configuration to make sure autogenerated files are not committed to source control. |
|
Configuration for Angular CLI. In this file you can set several defaults and also configure what files are included when your project is built. Check out the official documentation if you want to know more. |
|
|
|
End-to-end test configuration for Protractor,
used when running |
|
Basic documentation for your project, pre-filled with CLI command information. Make sure to enhance it with project documentation so that anyone checking out the repo can build your app! |
|
TypeScript compiler configuration for your IDE to pick up and give you helpful tooling. |
|
Linting configuration for TSLint together with
Codelyzer, used when running |