Documentation
Bind jqxDataTable to MySQL Database Using Spring MVC
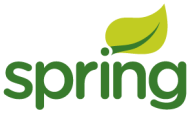
In this help topic you will learn how to bind a jqxDataTable to a MySQL database using the Spring Web MVC framework, which provides model-view-controller architecture and ready components that can be used to develop flexible and loosely coupled web applications. The MVC pattern results in separating the different aspects of the application (input logic, business logic, and UI logic), while providing a loose coupling between these elements.
1. Set Up a Database
Our goal is to populate a jqxDataTable from a MySQL database. In the example presented here, we will be using the Northwind database. To learn how to set it up, please follow Step 1 from the help topic Configure MySQL, Eclipse and Tomcat for Use with jQWidgets.
2. Create a New Project in Eclipse
In this tutorial, we will be using Eclipse IDE for Java EE Developers, version Luna.
- Go to File → New → Maven Project.
- In the New Maven Project window, make sure Create a simple
project (skip archetype selection) is unchecked and click Next.
- In the next window, click Add Archetype... and set Archetype
Group Id to org.apache.maven.archetypes, Archetype Artifact
Id to maven-archetype-webapp and Archetype Version
to 1.0. Then click OK.
- Select the newly added archetype and click Next.
- In the final window of the new project setup, set the Group Id
to com.jqwidgets, the Artifact Id to jqwidgets-spring
and the Package to war. Finally, click Finish.
3. Configure the Project
Add the necessary project dependencies in the file pom.xml
in the root
of the project:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemalocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.jqwidgets</groupId> <artifactId>jqwidgets-spring</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>jqwidgets-spring Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.codehaus.jackson</groupId> <artifactId>jackson-core-lgpl</artifactId> <version>1.9.9</version> </dependency> <dependency> <groupId>org.codehaus.jackson</groupId> <artifactId>jackson-mapper-lgpl</artifactId> <version>1.9.9</version> </dependency> </dependencies> <build> <finalName>jqwidgets-spring</finalName> </build> <properties> <spring.version>4.0.2.RELEASE</spring.version> </properties></project>
The file web.xml
, found in src\main\webapp\WEB-INF
, defines
everything about the application that the server needs to know. Configure it as
follows:
<?xml version="1.0" encoding="UTF-8" ?><web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemalocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>mvc-dispatcher</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>mvc-dispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping></web-app>
The <servlet> element declares the DispatcherServlet. When the DispatcherServlet
is initialized, the framework will try to load the application context from a file
named [servlet-name]-servlet.xml
located in the WEB-INF
directory. The <servlet-mapping> element specifies what URLs will be handled
by the DispatcherServlet.
The DispatcherServlet has to be created. Do so by right-clicking the WEB-INF
folder and selecting New → Other. Choose XML
File and name the file mvc-dispatcher-servlet.xml
. Add
the following as its content:
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemalocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> <context:component-scan base-package="com.jqwidgets" /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix"> <value>/WEB-INF/</value> </property> <property name="suffix"> <value>.jsp</value> </property> </bean> <mvc:resources mapping="/resources/**" location="/resources/" /> <mvc:annotation-driven /></beans>
4. Create the Model
We are going to populate a jqxDataTable with information about employees from the employees table in the Northwind database. That is why we need a Model class which describes an employee and their properties.
First, right-click Java Resources
and create a new Source Folder.
Set jqwidgets-spring as its Project name and src/main/java
as its Folder name. Next, create a new package in the folder named
com.jqwidgets
:
In the new package, create our Model class, Employee
. This class describes
an employee with their first name, last name, title and birth date with the following
code:
package com.jqwidgets;public class Employee { private String employeeId; private String firstName; private String lastName; private String title; private String birthDate; public Employee(String employeeId, String firstName, String lastName, String title, String birthDate) { setEmployeeId(employeeId); setFirstName(firstName); setLastName(lastName); setTitle(title); setBirthDate(birthDate); } public String getEmployeeId() { return employeeId; } public void setEmployeeId(String employeeId) { this.employeeId = employeeId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getBirthDate() { return birthDate; } public void setBirthDate(String birthDate) { this.birthDate = birthDate; }}
5. Create the Controller
The Controller is where the DispatcherServlet will delegate requests. It will retrieve
employee data from the database and store it in instances of the Employee
class. Create a new class, DataTableController
in the package com.jqwidgets
with the following content:
package com.jqwidgets;// Spring Frameworkimport org.springframework.stereotype.Controller;import org.springframework.ui.ModelMap;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RequestMethod;import org.springframework.web.bind.annotation.ResponseBody;// JDBCimport java.sql.*;// List and ArrayListimport java.util.List;import java.util.ArrayList;@Controller@RequestMapping("/datatable")public class DataTableController { @RequestMapping(method = RequestMethod.GET) public String dataTable(ModelMap model) { return "datatable"; } @RequestMapping(value = "/getEmployees", method = RequestMethod.POST) public @ResponseBody List<Employee> getEmployees() { List<Employee> employeeList = new ArrayList<Employee>(); try { // database connection // "jdbc:mysql://localhost:3306/northwind" - the database url of the form jdbc:subprotocol:subname // "root" - the database user on whose behalf the connection is being made // "abcd" - the user's password Connection dbConnection = DriverManager.getConnection( "jdbc:mysql://localhost:3306/northwind", "root", "abcd"); // retrieve necessary records from database Statement getFromDb = dbConnection.createStatement(); ResultSet employees = getFromDb .executeQuery("SELECT EmployeeID, FirstName, LastName, Title, BirthDate FROM employees"); // populate an ArrayList with the retrieved data while (employees.next()) { employeeList.add(new Employee( employees.getString("EmployeeID"), employees .getString("FirstName"), employees .getString("LastName"), employees .getString("Title"), employees .getString("BirthDate"))); } } catch (SQLException e) { e.printStackTrace(); } return employeeList; }}
6. Add the Necessary jQWidgets Scripts and Stylesheets to the Project
Create a resources
folder in src\main\webapp
and in it
add two more folders - js
and css
. Include in them all
(or only the necessary) jQWidgets files - the scripts (including jqxcore.js
and the specific widget files) in js
and the stylesheets (jqx.base.css
and any themes and associated images) in css
. Remember to include a
version of jQuery in js
, too.
7. Create the View (JSP)
The View is where the jqxDataTable with the employee data will be displayed. Create
a new JSP File in src\main\webapp\WEB-INF
and name
it datatable.jsp
. This page will serve as the View. Here is its content:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%><%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%><!DOCTYPE html><html lang="en"><head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>This example shows jqxDataTable binding to MySQL using Spring Web MVC.</title> <link rel="stylesheet" href="<c:url value="/resources/css/jqx.base.css" />" type="text/css" /> <link rel="stylesheet" href="<c:url value="/resources/css/jqx.arctic.css" />" type="text/css" /> <script type="text/javascript" src="<c:url value="/resources/js/jquery.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jqxcore.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jqxbuttons.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jqxscrollbar.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jqxdata.js" />"></script> <script type="text/javascript" src="<c:url value="/resources/js/jqxdatatable.js" />"></script> <script type="text/javascript"> $(document).ready(function () { var source = { dataType: 'json', dataFields: [{ name: 'firstName', type: 'string' }, { name: 'lastName', type: 'string' }, { name: 'title', type: 'string' }, { name: 'birthDate', type: 'date' }], id: 'employeeId', url: '${pageContext.request.contextPath}/datatable/getEmployees', type: 'POST', async: true }; var dataAdapter = new $.jqx.dataAdapter(source); $('#dataTable').jqxDataTable({ theme: 'arctic', width: 550, source: dataAdapter, columns: [{ text: 'First Name', dataField: 'firstName', width: 100 }, { text: 'Last Name', dataField: 'lastName', width: 100 }, { text: 'Title', dataField: 'title', width: 180 }, { text: 'Birth Date', dataField: 'birthDate', cellsFormat: 'd', align: 'right', cellsAlign: 'right' }] }); }); </script><script async src="https://www.googletagmanager.com/gtag/js?id=G-2FX5PV9DNT"></script><script>window.dataLayer = window.dataLayer || [];function gtag(){dataLayer.push(arguments);}gtag('js', new Date());gtag('config', 'G-2FX5PV9DNT');</script></head><body> <div id="dataTable"></div></body></html>
You will need to add the JSP Standard Tag Library to the project. Create a folder
named lib
in src\main\webapp\WEB-INF
and place the library's
JAR there. You can get it from this location: http://www.java2s.com/Code/Jar/j/Downloadjstl12jar.htm.
8. Run the Data Table Page
Before running the View, please make sure you have the Apache Tomcat server installed and configured as explained in Steps 5 and 6 from the tutorial Configure MySQL, Eclipse and Tomcat for Use with jQWidgets.
Right-click the project and select Run As → Run on Server. In the window that appears, select Tomcat v8.0 Server at localhost and click Finish.
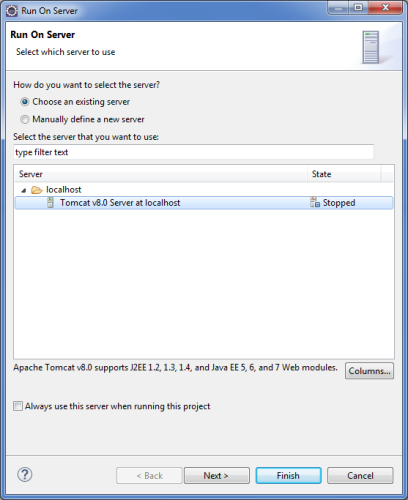
Go to http://localhost:8080/jqwidgets-spring/datatable to view the data table page:
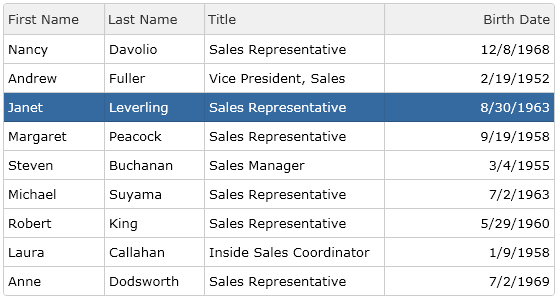
You can download the project as a WAR file from here: jqwidgets-spring.zip.