React UI Components Documentation
React WebPack & jQWidgets
You can download the final result here.
Steps:
- Create Your Project Folder Structure
- Configurate and Install All Required Packages
- Build the App
Step 1 - Create Your Project Folder Structure
The root folder contains a few configuration files and the src folder which holds the main content of the application.
/root
/src
/index.tsx
/App.tsx
/index.html
/package.json
/tsconfig.json
/webpack.config.js
Note: Structure may vary based on your application needs.
Step 2- Configurate and Install All Required Packages
package.json
{ "name": "jqwidgets-react-typescript-webpack", "author": "www.jqwidgets.com", "dependencies": { "@types/react": "^16.8.1", "@types/react-dom": "^16.0.11", "awesome-typescript-loader": "^5.2.1", "css-loader": "^2.1.0", "img-loader": "^3.0.1", "jqwidgets-scripts": "^7.0.0", "raw-loader": "^1.0.0", "react": "^16.8.0", "react-dom": "^16.8.0", "style-loader": "^0.23.1", "typescript": "^3.3.1", "webpack": "^4.28.3", "webpack-cli": "^3.2.1" }, "scripts": { "start": "webpack --config webpack.config.js" }}
tsconfig.json
{ "compilerOptions": { "outDir": "./dist/", "sourceMap": true, "noImplicitAny": true, "module": "commonjs", "target": "es5", "jsx": "react" }, "include": [ "./src/*" ]}
webpack.config.js
module.exports = { entry: { app: __dirname + '/src/index.tsx' }, output: { path: __dirname + '/dist', filename: '[name].bundle.js' }, resolve: { extensions: ['.ts', '.tsx', '.js', '.json'] }, module: { rules: [ { test: /\.tsx?$/, loader: 'awesome-typescript-loader' }, { test: /\.css$/, loaders: ['style-loader', 'css-loader'] }, { test: /\.(jpe?g|png|gif|svg)$/i, loaders: ['raw-loader', 'img-loader'] } ] }, externals: { 'react': 'React', 'react-dom': 'ReactDOM' }, mode: 'production' };
When all the config files are ready, it is time to install the packages:
npm install
Step 3- Build the App
index.html
<!DOCTYPE html><html> <head> <title id='Description'>React WebPack & jQWidgets</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link href="./node_modules/jqwidgets-scripts/jqwidgets/styles/jqx.base.css" type="text/css" rel="stylesheet" /> <script src="./node_modules/react/umd/react.development.js"></script> <script src="./node_modules/react-dom/umd/react-dom.development.js"></script> <script async src="https://www.googletagmanager.com/gtag/js?id=G-2FX5PV9DNT"></script><script>window.dataLayer = window.dataLayer || [];function gtag(){dataLayer.push(arguments);}gtag('js', new Date());gtag('config', 'G-2FX5PV9DNT');</script></head> <body> <div id="app"></div> <script src=./dist/app.bundle.js></script> </body> </html>
index.tsx
import * as React from 'react';import * as ReactDOM from 'react-dom'; import App from './App'; ReactDOM.render( <App />, document.getElementById('app') as HTMLElement);
App.tsx
import * as React from 'react'; import JqxBarGauge, { IBarGaugeProps } from 'jqwidgets-scripts/jqwidgets-react-tsx/jqxbargauge'; class App extends React.PureComponent<{}, IBarGaugeProps> { constructor(props: {}) { super(props); this.state = { tooltip: { formatFunction(value?: number | string): string { return 'Year: 2016 Price Index:' + value; }, visible: true }, values: [10, 20, 30, 40, 50] }; } public render() { return ( <JqxBarGauge width={600} height={600} max={60} colorScheme={'scheme02'} values={this.state.values} tooltip={this.state.tooltip} /> ); }} export default App;
Now when all files are populated, it is time to build the app:
npm start
A folder called dist will appear on root level. Inside will be our bundled application.
All that is left is to open index.html and enjoy your new app.
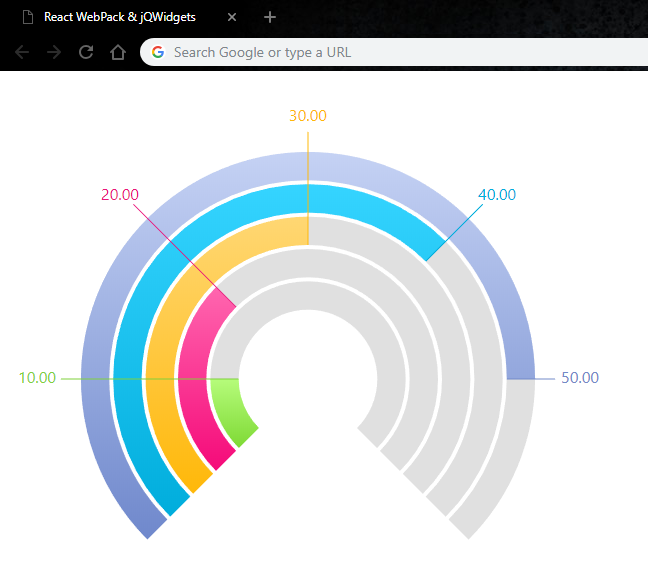